Product Design Lessons
Intro to Foundation | Lesson #80
Supe Up Your Sass With Mixins
Learn to make processing “methods” in Sass to quickly process variable information into CSS.
Little things matter, and less code is better. To that end we have Sass Mixins: bits of code that give back CSS with one little command. Done right, they can evaluate a bit of information and give you something more useful. In this lesson we'll write a mixin to make text readable on different color backgrounds — even if the background changes.
What mixins do
Mixins "return" information — that is, when you write their name in code, their results will take fill in the blank Here's a basic example:
@mixin doThis() { return 'red' }
This mixin has one job. Everywhere we write "doThis()
", Sass will write "red
" in CSS. Like so:
p { color: @include doThis(); }
aside { background-color: @include doThis(); }
div { border-color: @include doThis(); }
The result:
p { color: red; }
aside { background-color: red; }
div { border-color: red; }
Let's look at a more practical example. This next mixin will return white if the background is dark, or black, if the background is light. That automatic contrast is key to ensuring text is readable against its background. Sass has a function to test for lightness — but we need a mixin to judge if the background is light enough for dark text, or vice versa.
1. Create the mixin.
Creating a mixin is easy. Here's the code:
@mixin altColor ( $test-this-color ) { }
That "$test-this-color
" between the parenthesis is a variable that the mixin will examine. We don't know what it is, exactly, so we need a test.
2. Set up a test.
The lightness()
function determines how far from black a color is. It works on a scale of 0 (black) to 100 (white). Let's say that if $test-this-color
is lighter than 60%, then we want dark text. If it's darker than 60%, we want light text.
In Sass we write:
@if ( lightness($test-this-color) > 60% ) { return #000; }
In English: "If the given color is lighter than 60%, then send back #000 (black)."
3. Account for the opposite.
We can do the opposite with a similar bit of logic:
@else { return #fff; }
In English: "Otherwise send back #fff (white)."
The final code:
@mixin altColor ( $test-this-color ) {
@if ( lightness($test-this-color) > 60% ) {
return #000;
}
@else {
return #fff;
}
}
So how do we apply it? Like this:
$bg-color: #d0d0d0;
body {
background: $bg-color;
color: @include altColor($bg-color);
}
Because #d0d0d0 is lighter than 60%, the resulting CSS looks like this:
body { background: #d0d0d0; color: #000; }
That means if we darken $bg-color
then the body font color will adjust automatically.
But wait! Body uses $bg-color
and the mixin uses $test-this-color. Don't worry — $test-this-color
is something that only works inside of the mixin. So go ahead, send it any color:
@include altColor(#303030);
@include altColor(#93ab30);
@include altColor(navy);
That's one reason mixins are so handy. You can send any Sass variable or value their way, and they do their thing.
About the instructor
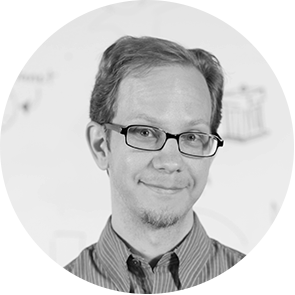
Ben Gremillion is a Design Writer at ZURB. He started his career in newspaper and magazine design, saw a digital future, and learned HTML in short order. He facilitates the ZURB training courses.
Product Design Lessons, Direct to Your Inbox
We're just getting started, so sign up and we'll keep you in the know with our product design lessons. No spam here.