Product Design Lessons
Intro to Foundation | Lesson #105
Clickable Prototypes for Those Who Hate Code
Learn useful jQuery functions explained in a practical way.
We have all been there. You are doing an awesome job pulling together a great design and then your client asks the dreaded question, “well how does this work.” You then start cobbling together a couple of sentences to try and describe how the interaction should look and how it should work. Simply put, it’s not the best approach.
Enter the idea of clickable prototypes, which is made even easier by front-end frameworks like ZURB Foundation. Sure, with Foundation you get a lot of things for free, but what happens when you want to start building out some basic interactivity that goes beyond the built in components?
The usual route is to pair up with a developer and have them help you write those interactive bits. Today I am hoping to shed some light on simple patterns that, dare I say, can help you even go as far as copy and pasting your way to interactive bliss.
I can’t promise that we are at code-zero, but by the end of this article you have 3 of the most common patterns that should handle about 95% of simple interaction you might run across.
Getting Started with IDs
The first thing you want to do is add an ID to the html element that you want to add interaction to. In this case, let’s just create a simple button adding our ID #css-power
.
<a id=”css-power” class=”button”>Behold the Interactive Power of CSS</a>
Some say that it is bad practice to add IDs to elements or to use them in CSS. I won’t argue either way, but setting IDs are critical when we are using JavaScript. This is because you want a specific element to trigger the interaction, not a whole class of them.
Is the DOM Ready?
The second thing we need to do is wait for DOM Ready. Why do that? Simple. We want all of our other JS Components such as Orbit, Top-bar, and Off-canvas to be loaded and ready to go before we go about changing things.
$(document).ready( function() {
// we will add our interactive code here
});
So what exactly is going on here?
First we are using jQuery by using the $
shortcut and we are passing in the document object. Not sure what the document object is? No problem, we are just waiting for it to be ready, hence, the ready()
function that is called.
This is doubly confusing since we seem to be passing another function into this function. Well confusing or not, this is the whacky world of JavaScript programming and jQuery.
To break this down further, we are using jQuery to tell us when the document object is ready. Once that happens whatever is passed in our “function” is then run in order.
Pattern 1 - Adding, Removing + Toggling CSS FTW
Now let's get down to some simple JavaScript patterns that you can mix and match to add some basic interaction and start showing states live in your html prototypes.
Show, don’t tell as they say.
If you understand basic CSS you should understand jQuery as well. That is because both share the same CSS selector patterns.
To illustrate that we can type the following into the developer console in Chrome or Firefox.
$(“#css-power”);
You should be presented with something like:
<a class="button" id="css-power">Behold the Interactive Power of CSS</a>
Awesome, just want we wanted. We'll use this button for our example.
Side note: You can type all sorts of commands using jQuery into the console and it is a great way to experiment and make sure that you are selecting the correct objects.
Our next step is to add some CSS so that we track our changes visually. To do that we need to add an .active
class to our CSS/Scss.
.button.active {
border: solid 4px #FA7CA7;
}
Now that we have a class to visualize the change, we can trigger it using jQuery directly from the console.
$(“#css-power”).addClass(“active”);
Now let’s put it all together and make our button trigger the action. We are able to do this using the on()
function.
$(document).ready(function() {
$("#css-power").on("click", function() {
$("#css-power").addClass("active");
});
});
This can be a bit overwhelming if you hate code, so let’s break that down step-by-step:
- Wait for DOM to be ready.
- Add a handler to the
#css-power
element usingon()
and set it to ‘click’. This does exactly what it sounds like, it waits until that element is clicked and then runs the function. - Use
addClass()
to add the CSS class.active
to our element#css-power
.
And voila, we click the button the class is applied.
Check out the example ⇨
Two other functions that also come in handy are:
Every Motion UI effect is customizable. Let's replace the stock fade animation with a custom hinge animation.
- removeClass remove a class from the element
- toggleClass turn a class on and off automatically
Pattern 2: Hide and Show
Another common pattern in prototypes is showing and hiding elements. One example is a form where you want to display additional information based on what answer is given.
<label>What is your favorite Responsive Framework</label>
<input type="radio" name="framework" value="Foundation" id="framework-foundation">
<label for="framework-foundation">ZURB Foundation</label>
<input type="radio" name="framework" value="Other" id="framework-other">
<label for="framework-other">Other</label>
And in this example we want to show an additional panel if they select Foundation.
<div class="panel" id="foundation-drilldown">
<label for="how">We also love ZURB Foundation! Could you tell us how you use it?</label>
<input type="text" name="how" placeholder="How did you find out?">
</div>
The first step here is to hide our additional information panel since it is visible by default. This is easily accomplished using Foundation’s visibility class .hide
.
<div class="hide panel" id="foundation-drilldown">
<label for="how">We also love ZURB Foundation! Could you tell us how you use it?</label>
<input type="text" name="how" placeholder="How did you find out?">
</div>
Now that the panel is hidden from view, we can start by adding code to show the panel when the #framework-foundation radio button is clicked.
$("#framework-foundation").on("click", function() {
$("#foundation-drilldown").removeClass("hide");
});
This is very similar to Pattern 1, with the exception of that we are removing the class from a different element called #foundation-drilldown which is our hidden panel. Now that we can show the panel, but we also need to hide it.
$("#framework-other").on("click", function() {
$("#foundation-drilldown").addClass("hide");
});
Check out the example ⇨
Pattern 3: Select One
The final pattern is what I like to call select one. The way to think about this is a component that acts like a radio button. This can be a list of tags, buttons or other elements where the key interaction is that only one can be selected at any given time.
<p>What is your favorite TV Show?</p>
<ul class="inline-list">
<li><a class="tv-button button active" id="show-once">Once Upon a Time</a></li>
<li><a class="tv-button button" id="show-person">Person of Interest</a></li>
<li><a class="tv-button button" id="show-robot">Mr. Robot</a></li>
</ul>
In building a prototype you might show this state by setting one to .active
, as I have above. Next you would need to build out the CSS to represent the visual interaction.
.tv-button {
@include button($button-tny, $secondary-color);
}
.tv-button.active {
border-bottom: solid 4px $primary-color;
padding-bottom: $button-tny - rem-calc(4);
}
This is a pretty common way of approaching things. Now let’s take this one step further and make it interactive.
This pattern builds on our first two patterns but has a slight variation.
$(".tv-button").on("click", function() {
$(".tv-button").removeClass("active");
$(this).addClass("active");
});
Here we are using the .tv-button
class instead of an ID. This allows us to set the click behavior of all of our buttons at the same time. Next we are going to remove the .active
class of all of our buttons at the same time, including the one we just clicked.
What is “$(this)”
?
The last part is a little bit tricky and adds a new keyword called this. When paired with jQuery $(this) is a reference to the element that is triggering the on(“click”)
function. This represents that specific element and allows us to directly turn the .active
class on by using addClass(“active”)
.
Check out the example ⇨
Next Steps
Using jQuery for clickable prototypes can quickly add interactivity and a level of clarity to how things will work. These 3 jQuery interactions will help you prototype simple interactions. We’re just scratching the surface here and hope these simple explanations help you love code as much as I do.
About the instructor
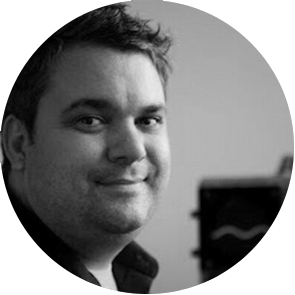
James Stone ( @jamesstoneco ) wrote ZURB Foundation Blueprints and is a top contributor to ZURB Foundation and is an Adjunct Professor at Penn State. He's created tons of video tutorials and writes helpful blogs and coding tips. He offers consulting, development, and support services at jamesstone.com.
Product Design Lessons, Direct to Your Inbox
We're just getting started, so sign up and we'll keep you in the know with our product design lessons. No spam here.